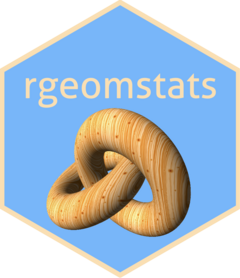
Class for the Manifold of Symmetric Positive Definite Matrices
Source:R/spd-matrices.R
SPDMatrices.Rd
Class for the manifold of symmetric positive definite (SPD) matrices.
See also
Other symmetric positive definite matrix classes:
SPDMatrix()
Super classes
rgeomstats::PythonClass
-> rgeomstats::Manifold
-> rgeomstats::OpenSet
-> SPDMatrices
Methods
Inherited methods
rgeomstats::PythonClass$get_python_class()
rgeomstats::PythonClass$set_python_class()
rgeomstats::Manifold$belongs()
rgeomstats::Manifold$is_tangent()
rgeomstats::Manifold$random_point()
rgeomstats::Manifold$random_tangent_vec()
rgeomstats::Manifold$regularize()
rgeomstats::Manifold$set_metric()
rgeomstats::Manifold$to_tangent()
rgeomstats::OpenSet$projection()
Method new()
The SPDMatrices
class constructor.
Usage
SPDMatrices$new(n, ..., py_cls = NULL)
Arguments
n
An integer value specifying the number of rows and columns of the matrices.
...
Extra arguments to be passed to parent class constructors. See
OpenSet
andManifold
classes.py_cls
A Python object of class
SPDMatrices
. Defaults toNULL
in which case it is instantiated on the fly using the other input arguments.
Examples
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3
}
Method cholesky_factor()
Computes Cholesky factor for a symmetric positive definite matrix.
Method differential_cholesky_factor()
Computes the differential of the Cholesky factor map.
Arguments
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method expm()
Computes the matrix exponential for a symmetric matrix.
Arguments
mat
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices.
Examples
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$expm(diag(-1, 3))
}
Method differential_exp()
Computes the differential of the matrix exponential.
Arguments
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method inverse_differential_exp()
Computes the inverse of the differential of the matrix exponential.
Arguments
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method logm()
Computes the matrix logarithm of an SPD matrix.
Arguments
mat
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices.
Examples
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$logm(diag(1, 3))
}
Method differential_log()
Computes the differential of the matrix logarithm.
Arguments
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method inverse_differential_log()
Computes the inverse of the differential of the matrix logarithm.
Arguments
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method powerm()
Computes the matrix power of an SPD matrix.
Arguments
mat
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices.
power
A numeric value or vector specifying the desired power(s).
Returns
A numeric array of the same shape as mat
storing the
corresponding matrix powers computed as: $$A^p = \exp(p \log(A)).$$
If power
is a vector, a list of such arrays is returned.
Examples
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$powerm(diag(1, 3), 2)
}
Method differential_power()
Computes the differential of the matrix power function.
Arguments
power
An integer value specifying the desired power.
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Method inverse_differential_power()
Computes the inverse of the differential of the matrix power function.
Arguments
power
An integer value specifying the desired power.
tangent_vec
A numeric array of shape \([\dots \times n \times n]\) specifying one or more symmetric matrices at corresponding base points.
base_point
A numeric array of shape \([\dots \times n \times n]\) specifying one or more SPD matrices specifying base points for the input tangent vectors.
Examples
## ------------------------------------------------
## Method `SPDMatrices$new`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3
}
## ------------------------------------------------
## Method `SPDMatrices$cholesky_factor`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$cholesky_factor(A)
}
## ------------------------------------------------
## Method `SPDMatrices$differential_cholesky_factor`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$differential_cholesky_factor(diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$expm`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$expm(diag(-1, 3))
}
## ------------------------------------------------
## Method `SPDMatrices$differential_exp`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$differential_exp(diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$inverse_differential_exp`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$inverse_differential_exp(diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$logm`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$logm(diag(1, 3))
}
## ------------------------------------------------
## Method `SPDMatrices$differential_log`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$differential_log(diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$inverse_differential_log`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$inverse_differential_log(diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$powerm`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
spd3$powerm(diag(1, 3), 2)
}
## ------------------------------------------------
## Method `SPDMatrices$differential_power`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$differential_power(2, diag(1, 3), A)
}
## ------------------------------------------------
## Method `SPDMatrices$inverse_differential_power`
## ------------------------------------------------
if (reticulate::py_module_available("geomstats")) {
spd3 <- SPDMatrix(n = 3)
V <- cbind(
c(sqrt(2) / 2, -sqrt(2) / 2, 0),
c(sqrt(2) / 2, sqrt(2) / 2, 0),
c(0, 0, 1)
)
A <- V %*% diag(1:3) %*% t(V)
spd3$inverse_differential_power(2, diag(1, 3), A)
}